Overview
This is a light weight api server aimed at making it easy to create, store and serve geospatial data to other webmapping applications. The core api server is written in GoLang and uses three Go packages: Gorilla Mux , Gorilla WebSocket , go.geojson and Bolt.
Features
Installation
Install GoLang
Follow the guide to install GoLang on your computer.
Get the Code
With GoLang installed, we will have to get the latest GoSpatial source code. We can do this simply cloning the repository:
$ git clone https://github.com/sjsafranek/gospatial.git
Installing Dependencies
We will now need to aquire the three Go packages (Gorilla Mux , Gorilla WebSocket and Bolt). There is a simple shell script provided in the repositories main directory that will go get
these packages and setup the working directory:
$ cd gospatial
$ ./install.sh
Building from source
Finally we can compile from our source:
$ make install
We are now ready to run the server!
Quickstart
Start the server
Start server with the by executing the binary file produced in the bin directory:
stefan@stefan:~/Repos/gospatial$ ./bin/gospatial
______ __ _ __
/ ____/___ _________ ____ _/ /_(_)___ _/ /
/ / __/ __ \/ ___/ __ \/ __ '/ __/ / __ '/ /
/ /_/ / /_/ (__ ) /_/ / /_/ / /_/ / /_/ / /
\____/\____/____/ .___/\__,_/\__/_/\__,_/_/
/_/
2016/04/16 13:04:28 Authkey: su
2016/04/16 13:04:28 Database: bolt
2016/04/16 13:04:28 Profiling happens on port 6060...
2016/04/16 13:04:28 Magic happens on port 8080...
Options
Option | Default | Description |
---|---|---|
-db | bolt.db | Specifies what database file to use |
-p | 8080 | Specifies the server port |
-s | su | Specifies the superuser key for management routes |
-c | Gets port, database, and superuser key from config json file (produced by ./setup) | |
-v | Prints the app version |
Generate settings.json
To create a settings.json (server config) run the following command
stefan@stefan:~/Repos/gospatial$ ./setup
server port: 8888
database: bolt
authkey: testing
This will create a file called settings.json
{
"port": 8888,
"db": "bolt.db",
"authkey": "testing"
}
Troubleshooting
You are bound to run into problems. Please review the following hints for troubleshooting. Of course, you're welcome to contact us if you're unable to resolve.
Customer Routes
View layers
GET /api/v1/layers?apikey=<apikey>
Returns json containing customers datasources
- apikey - customer apikey
{
"apikey": "TIJ3ZzsIWG8k",
"datasources": [
"b3db766e432842398ea1e9364acb5ad6"
]
}
Create layer
POST /api/v1/layer?apikey=<apikey>
Creates a new datasource layer and returns datasource uuid
- apikey - customer apikey
{
"datasource": "b2e10ca0d3bf466d90a427671ad00f37",
"status": "ok"
}
View layer
GET /api/v1/layer/{ds}?apikey=<apikey>
Returns GeoJSON for requested datasource layer
- ds (in url) - datsource layer uuid
- apikey - customer apikey
{
"type": "FeatureCollection",
"features": [
{
"type": "Feature",
"geometry": {
"coordinates": [10,-10],
"type": "Point"
},
"properties": {
"name": "Our House"
}
},
{
"type": "Feature",
"geometry": {
"coordinates": [
[-101.01,-100.01],
[100.01,101.01],
[-110.1,-10.1],
],
"type": "LineString"
},
"properties": {
"name": "Middle of the Street"
}
}
]
}
Delete layer
DELETE /api/v1/layer{ds}?apikey=<apikey>
Deletes a datasource layer and removes it from customer
- ds (in url) - datsource layer uuid
- apikey - customer apikey
{
"status":"ok",
"datasource":"8665c8ee0cc44b56848acfb55302e3a9",
"result":"datasource deleted"
}
Create feature
POST /api/v1/layer/{ds}/feature?apikey=<apikey>
Adds GeoJSON feature to datasource layer
- ds (in url) - datsource layer uuid
- apikey
{
"geometry": {
"type": "Point",
"coordinates": [0, 0]
},
"properties": {
"name": "Center"
}
}
{
"status": "ok",
"datasource": "b2e10ca0d3bf466d90a427671ad00f37",
"result":"feature added"
}
View feature
GET /api/v1/layer/{ds}/feature{k}?apikey=<apikey>
Adds GeoJSON feature to datasource layer
- ds (in url) - datsource layer uuid
- k (in url) - index of feature
- apikey - customer apikey
{
"geometry": {
"coordinates": [0, 0],
"type": "Point"
},
"type": "Feature",
"properties": {
"name": "Center"
}
}
Superuser Routes
Add Customer
POST /management/customer?authkey=<superuserkey>
Creates a new customer and returns customer apikey
- authkey - superuser apikey
{
"apikey": "TIJ3ZzsIWG8k",
"status": "ok",
"result": "new customer created"
}
Share Layer
PUT /api/v1/layer/{ds}?apikey=<apikey>&authkey=<superuserkey>
Shares datasource layer with another customer
- ds (in url) - datsource layer uuid
- apikey - customer apikey
- authkey - superuser apikey
{
"status": "ok",
"datasource": "b2e10ca0d3bf466d90a427671ad00f37",
"result":"layer shared"
}
Server profile
GET /management/profile?authkey=<superuserkey>
Returns basic server info such as uptime
- authkey - superuser apikey
{
"num_cores": 1,
"registered": "2016-03-18T06:48:12.519759979Z",
"status": "standard",
"uptime": 56642.641125179
}
Views
Map Client
GET /map/{ds}?apikey=<superuserkey>
Mobile friendy map client using the Leaflet mapping library. Easy to use interface for viewing and submitting geospatial features. This view is touch screen supported.
- ds (in url) - datsource layer uuid
- apikey - customer apikey
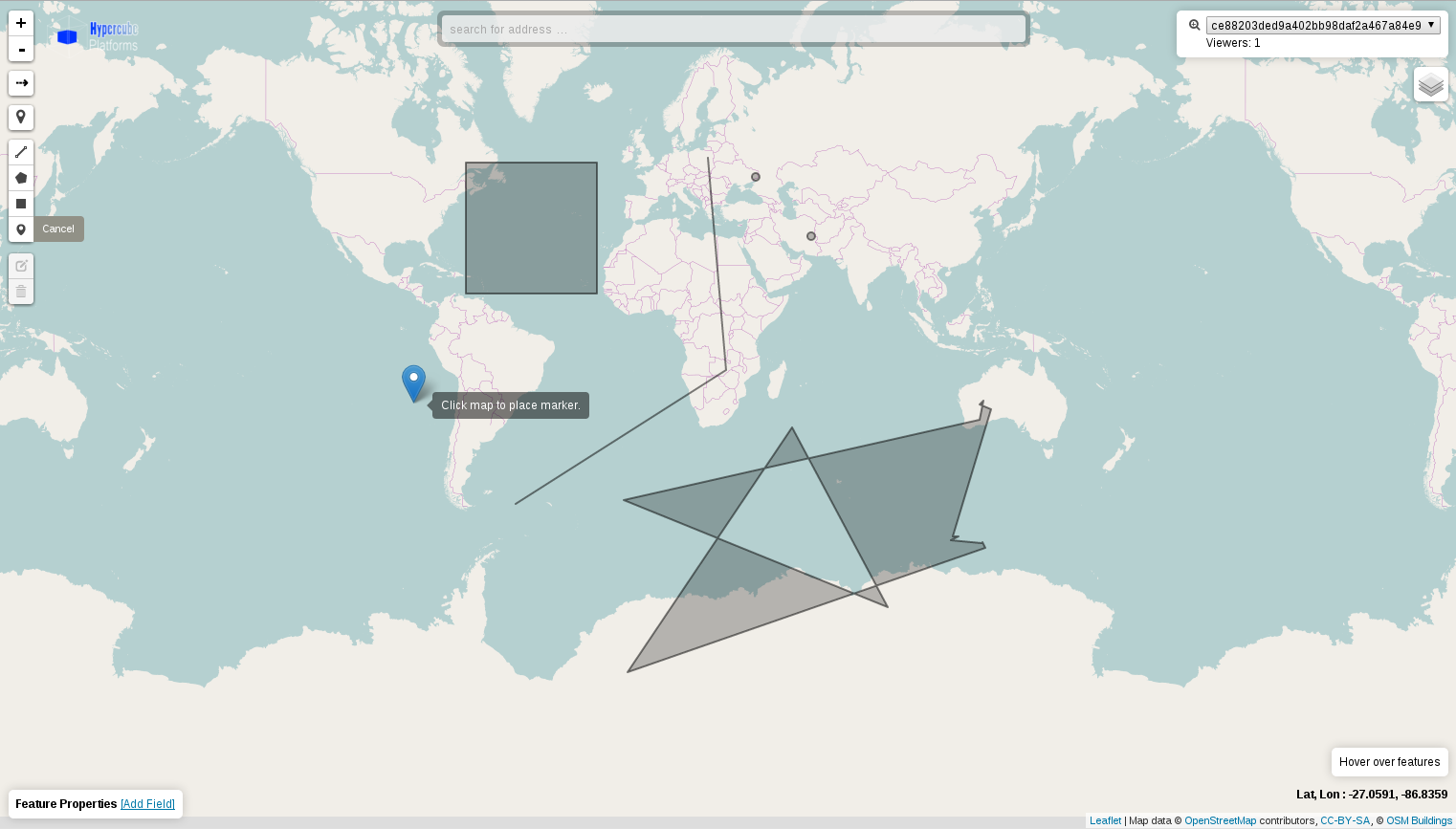
Management
GET /management?apikey=<superuserkey>
Simple interface for creating and managing your datasources. Users are able to create and delete layers, and link basemap layers
- apikey - customer apikey
Command line tools
gospatial_cmd
Command line tool for backend operations
Options
Option | Default | Description |
---|---|---|
-db | bolt.db | Specifies what database file to use |
Methods
Method | Arg | Arg | Description |
---|---|---|---|
ls | Lists all datasources in database | ||
export | datasource id | Exports datasource to GeoJSON file | |
import | *.shp | Imports shapefile into database | |
import | *.geojson | Imports geojson file into database | |
create | datasource | Creates new datasource | |
create | customer | Creates new customer | |
assign | datasource id | customer key | Assigns datasource to customer |